Archive Old Gmail Messages Automatically
This Google Script will scan the inbox of your Gmail and archive any message that you’ve read (seen) and is older than a month. It skips messages that are either starred or marked with a particular label like toReply.
function archiveInbox() {
var query = 'label:inbox is:read older_than:30d -in:starred -label:toreply';
var batchSize = 100;
while(GmailApp.search(query, 0, 1).length == 1) {
GmailApp.moveThreadsToArchive(GmailApp.search(query, 0, batchSize));
}
}
Here's an alternate way to deal with the same issue. It checks for individual messages in a Gmail thread before moving them to the Archive.
function cleanInbox() {
var threads = GmailApp.getInboxThreads();
for (var i = 0; i < threads.length; i++) {
var thread=threads[i];
if (!thread.hasStarredMessages() && !thread.isUnread()) {
GmailApp.moveThreadToArchive(threads[i]);
}
}
}
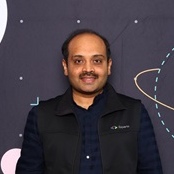
Amit Agarwal
Google Developer Expert, Google Cloud Champion
Amit Agarwal is a Google Developer Expert in Google Workspace and Google Apps Script. He holds an engineering degree in Computer Science (I.I.T.) and is the first professional blogger in India.
Amit has developed several popular Google add-ons including Mail Merge for Gmail and Document Studio. Read more on Lifehacker and YourStory