Simple PHP Templates with Placeholders in Curly Braces
This is a simple PHP based template where the variable fields in the template are enclosed in double parenthesis and the actual values are passed in a single array. The entire substitution happens in one step using preg_replace.
If your template string is long, you can either put that in a separate PHP file (and use the include function) or simply use multi-line strings with the Heredoc syntax (delimited by <<<
).
<?php
$template = "I am {{name}}, and I work for {{company}}. I am {{age}}.";
# Your template tags + replacements
$replacements = array(
'name' => 'Jeffrey',
'company' => 'Envato',
'age' => 27
);
function bind_to_template($replacements, $template) {
return preg_replace_callback('/{{(.+?)}}/',
function($matches) use ($replacements) {
return $replacements[$matches[1]];
}, $template);
}
// I am Jeffrey, and I work for Envato. I am 27.
echo bind_to_template($replacements, $template);
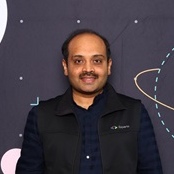
Amit Agarwal
Google Developer Expert, Google Cloud Champion
Amit Agarwal is a Google Developer Expert in Google Workspace and Google Apps Script. He holds an engineering degree in Computer Science (I.I.T.) and is the first professional blogger in India.
Amit has developed several popular Google add-ons including Mail Merge for Gmail and Document Studio. Read more on Lifehacker and YourStory