Update Google Contacts with Google Apps Script
This Google Apps Script project will allow your existing contacts to update their Google Contacts entries using a simple online form. The HTML form is published using Apps Script’s HtmlService.
function go() {
var emailNAME = ScriptProperties.getProperty('NAME');
var googleGROUP = ContactsApp.getContactGroup(ScriptProperties.getProperty('GROUP'));
if (googleGROUP) {
var emailSUBJECT = 'Your contact information';
var myContacts = googleGROUP.getContacts();
for (i = 0; i < myContacts.length; i++) {
var email = myContacts[i].getPrimaryEmail();
var ID = myContacts[i].getId();
ID = ID.substr(ID.lastIndexOf('/') + 1);
var emailBody =
'Hi,<br /><br />' +
'Would you please take a moment and update your contact information in my address book. <br /><br />' +
"Please <a href='" +
ScriptApp.getService().getUrl() +
'?' +
Utilities.base64Encode(ID + '#' + email) +
"'>click here</a> and fill-in the required details." +
'Your information will be directly added to my Google Contacts.' +
'<br /><br />Thanks,<br />' +
emailNAME;
var emailTEXT =
'Hi,\n\n' +
'Would you please take a moment and update your contact information in my address book. \n\n' +
ScriptApp.getService().getUrl() +
'?' +
Utilities.base64Encode(ID + '#' + email) +
'\n\nJust click the URL, fill-in the form and your details will be directly added to my Google Contacts.' +
'\n\nThanks,\n' +
emailNAME;
GmailApp.sendEmail(email, emailSUBJECT, emailTEXT, {
htmlBody: emailBody,
name: emailNAME,
});
}
}
}
function doGet() {
var html = HtmlService.createTemplateFromFile('form').evaluate();
html.setTitle('Google Contacts - Digital Inspiration');
return html;
}
function labnolGetContact(q) {
var contact = {};
contact.FOUND = 0;
try {
var id = Utilities.base64Decode(q);
var email = '';
for (var i = 0; i < id.length; i++) {
email += String.fromCharCode(id[i]);
}
var contactID = email.substr(0, email.indexOf('#'));
email = email.substr(email.indexOf('#') + 1);
var c = ContactsApp.getContact(email);
if (c) {
var originalID = c.getId();
originalID = originalID.substr(originalID.lastIndexOf('/') + 1);
if (contactID != originalID) return contact;
contact.FOUND = 1;
if (c.getFullName().length) contact.FULL_NAME = c.getFullName();
if (c.getEmails(ContactsApp.Field.HOME_EMAIL).length)
contact.HOME_EMAIL = c.getEmails(ContactsApp.Field.HOME_EMAIL)[0].getAddress();
if (c.getAddresses(ContactsApp.Field.HOME_ADDRESS).length) {
contact.HOME_ADDRESS = c.getAddresses(ContactsApp.Field.HOME_ADDRESS)[0].getAddress();
contact.HOME_ADDRESS = contact.HOME_ADDRESS.replace(/\n/g, ', ');
}
if (c.getPhones(ContactsApp.Field.MOBILE_PHONE).length)
contact.MOBILE_PHONE = c.getPhones(ContactsApp.Field.MOBILE_PHONE)[0].getPhoneNumber();
if (c.getIMs(ContactsApp.Field.SKYPE).length) contact.SKYPE = c.getIMs(ContactsApp.Field.SKYPE)[0].getAddress();
if (c.getUrls(ContactsApp.Field.BLOG).length) contact.BLOG = c.getUrls(ContactsApp.Field.BLOG)[0].getAddress();
if (c.getDates(ContactsApp.Field.BIRTHDAY).length) {
var months = [
0,
ContactsApp.Month.JANUARY,
ContactsApp.Month.FEBRUARY,
ContactsApp.Month.MARCH,
ContactsApp.Month.APRIL,
ContactsApp.Month.MAY,
ContactsApp.Month.JUNE,
ContactsApp.Month.JULY,
ContactsApp.Month.AUGUST,
ContactsApp.Month.SEPTEMBER,
ContactsApp.Month.OCTOBER,
ContactsApp.Month.NOVEMBER,
ContactsApp.Month.DECEMBER,
];
contact.BIRTHDAY =
months.indexOf(c.getDates(ContactsApp.Field.BIRTHDAY)[0].getMonth().toString()) +
'/' +
c.getDates(ContactsApp.Field.BIRTHDAY)[0].getDay() +
'/' +
c.getDates(ContactsApp.Field.BIRTHDAY)[0].getYear();
}
}
return contact;
} catch (e) {
return contact;
}
}
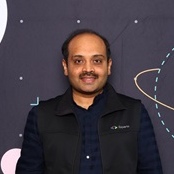
Amit Agarwal
Google Developer Expert, Google Cloud Champion
Amit Agarwal is a Google Developer Expert in Google Workspace and Google Apps Script. He holds an engineering degree in Computer Science (I.I.T.) and is the first professional blogger in India.
Amit has developed several popular Google add-ons including Mail Merge for Gmail and Document Studio. Read more on Lifehacker and YourStory