Insert Inline Images in Gmail with Apps Script
The GmailApp service of Google Apps Script lets you easily read and process email messages from Gmail. You can use this to forward Gmail messages, to save email to your Gmail Drive in HTML or PDF formats, Mail merge, or even for transferring emails from one Gmail account to another email account.
While GmailApp offers simple methods like sendEmail and forward() to forward existing Gmail messages, you need to update the HTML Mail if the message body contains inline images. This is only required for inline images and not externally hosted image files that have been embedded into Gmail.
function sendEmailMessage() {
var messageID = 'ABC'; // Put the Gmail Message ID here
var message = GmailApp.getMessageById(messageID);
var options = getInlineImages(message);
GmailApp.sendEmail(Session.getActiveUser().getEmail(), message.getSubject(), '', options);
}
function getInlineImages(message) {
var body = message.getBody();
var attachments = message.getAttachments();
var rawc = message.getRawContent();
var inlineImages = {};
var imgTags = body.match(/<img[^>]+>/g) || []; // all image tags, embedded or by url
for (var i = 0; i < imgTags.length; i++) {
var realattid = imgTags[i].match(/realattid=(.*?)&/i); // extract the image cid if embedded
if (realattid) {
// image is inline and embedded
var cid = realattid[1];
var imgTagNew = imgTags[i].replace(/src="[^\"]+\"/, 'src="cid:' + cid + '"'); // replace the long-source with just the cid
body = body.replace(imgTags[i], imgTagNew); // update embedded image tag in message body
var b64c1 = rawc.lastIndexOf(cid) + cid.length + 3; // first character in image base64
var b64cn = rawc.substr(b64c1).indexOf('--') - 3; // last character in image base64
var imgb64 = rawc.substring(b64c1, b64c1 + b64cn + 1); // is this fragile or safe enough?
var imgblob = Utilities.newBlob(Utilities.base64Decode(imgb64), 'image/jpeg', cid); // decode and blob
inlineImages[cid] = imgblob;
}
}
return {
htmlBody: body,
inlineImages: inlineImages,
attachments: attachments,
};
}
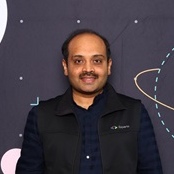
Amit Agarwal
Google Developer Expert, Google Cloud Champion
Amit Agarwal is a Google Developer Expert in Google Workspace and Google Apps Script. He holds an engineering degree in Computer Science (I.I.T.) and is the first professional blogger in India.
Amit has developed several popular Google add-ons including Mail Merge for Gmail and Document Studio. Read more on Lifehacker and YourStory