RegEx - Extract Video ID from YouTube URLs
The regular expression will extract the video ID from any YouTube URL and it works with shortened URLs youtu.be
and embed URLs that in the format youtube.com/embed
.
function extractVideoID(url) {
var regExp = /^.*((youtu.be\/)|(v\/)|(\/u\/\w\/)|(embed\/)|(watch\?))\??v?=?([^#\&\?]*).*/;
var match = url.match(regExp);
if (match && match[7].length == 11) {
return match[7];
} else {
alert('Could not extract video ID.');
}
}
Another alternative that will extract the video ID as well as the playlist ID from YouTube URLS:
var id = url.match('v=([a-zA-Z0-9_-]+)&?')[1];
var list = url.match('list=([a-zA-Z0-9-_]+)&?');
list = list ? list[1] : '';
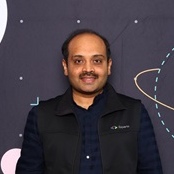
Amit Agarwal
Google Developer Expert, Google Cloud Champion
Amit Agarwal is a Google Developer Expert in Google Workspace and Google Apps Script. He holds an engineering degree in Computer Science (I.I.T.) and is the first professional blogger in India.
Amit has developed several popular Google add-ons including Mail Merge for Gmail and Document Studio. Read more on Lifehacker and YourStory