Monitor Files in Google Drive with Apps Script
The Drive Activity Report tracks changes to the files and documents in your Google Drive and send the list in an email every midnight. It also logs the changes in a Google Sheet.
It internally uses the DriveApp.search() method to find these files that have the modifiedDate property greater than the 24 hour period.
function driveActivityReport() {
var ss = SpreadsheetApp.getActiveSpreadsheet();
var sheet = ss.getActiveSheet();
// Get the spreadsheet time zone
var timezone = ss.getSpreadsheetTimeZone();
// Find files modified in the last 24 hours
var today = new Date();
var oneDayAgo = new Date(today.getTime() - 1 * 24 * 60 * 60 * 1000);
var startTime = oneDayAgo.toISOString();
// The magic search expression
var search = '(trashed = true or trashed = false) and (modifiedDate > "' + startTime + '")';
var files = DriveApp.searchFiles(search);
// Loop through all the files in the search results
while (files.hasNext()) {
var file = files.next();
var fileName = file.getName();
var fileURL = file.getUrl();
var dateCreated = Utilities.formatDate(file.getDateCreated(), timezone, 'yyyy-MM-dd HH:mm');
sheet.appendRow([dateCreated, fileName, fileURL]);
}
}
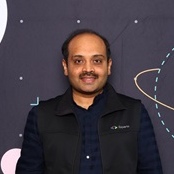
Amit Agarwal
Google Developer Expert, Google Cloud Champion
Amit Agarwal is a Google Developer Expert in Google Workspace and Google Apps Script. He holds an engineering degree in Computer Science (I.I.T.) and is the first professional blogger in India.
Amit has developed several popular Google add-ons including Mail Merge for Gmail and Document Studio. Read more on Lifehacker and YourStory