Blogger to WordPress Redirection
The Blogger to WordPress tutorial uses this PHP snippet to redirect visitors on the Blogger blog to the corresponding post on the WordPress site.
When a visitor is redirected from Blogger to WordPress, the blogger slug is contained in the query parameter string. On the WordPress side, this string is parsed and matched against the “blogger_permalink” custom fields on WordPress. If a match if found, the visitor is redirected to the corresponding WordPress post using a 301 redirect.
/* The Blogger URL passes the permlink in the query string */
function labnol_blogger_query_vars_filter( $vars ) {
$vars[] = "blogger";
return $vars;
}
add_filter('query_vars', 'labnol_blogger_query_vars_filter');
/* We take the blogger parameter and map it to the right WordPress post */
function labnol_blogger_template_redirect() {
global $wp_query;
$blogger = $wp_query->query_vars['blogger'];
if ( isset ( $blogger ) ) {
wp_redirect( labnol_get_wordpress_url ( $blogger ) , 301 );
exit;
}
}
add_action( 'template_redirect', 'labnol_blogger_template_redirect' );
function labnol_get_wordpress_url($blogger) {
/* Extract the blogger slug from the full blogspot.com url */
if ( preg_match('@^(?:https?://)?([^/]+)(.*)@i', $blogger, $url_parts) ) {
/* Query the WordPress database to find matching posts */
$query = new WP_Query ( array (
"meta_key" => "blogger_permalink", "meta_value" => $url_parts[2] ) );
/* if a match is found, get the permalink of the WordPress post */
if ($query->have_posts()) {
$query->the_post();
$url = get_permalink();
}
wp_reset_postdata();
}
/* If permalink is not found, return the blog home page url */
return $url ? $url : home_url();
}
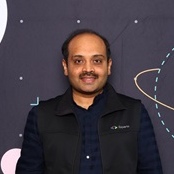
Amit Agarwal
Google Developer Expert, Google Cloud Champion
Amit Agarwal is a Google Developer Expert in Google Workspace and Google Apps Script. He holds an engineering degree in Computer Science (I.I.T.) and is the first professional blogger in India.
Amit has developed several popular Google add-ons including Mail Merge for Gmail and Document Studio. Read more on Lifehacker and YourStory