Create Google Contacts from Google Voice Mail
Google Voice sends you an email notification where there’s a missed call or a voicemail for you. The email includes the caller’s name, the caller’s phone number and, in case of voicemails, the text transcript of the message. The message also includes a link to download the Google Voice MP3.
The Google Scripts scans all your Google Voice emails, parses the content uses regex and creates a new Google contact. The transcript of the voice message is set to the notes field of the new Google contact.
Link: Save Google Voice Messages to Google Drive
// Search Google Voicemail emails
function searchGoogleVoiceEmails() {
var threads = GmailApp.search('from:voice-noreply@google.com', 0, 100);
for (var t = 0; t < threads.length; t++) {
var response = extractVoicemail_(threads[t].getMessages()[0]);
if (response) {
createContact_(response);
}
}
}
// Extract the caller's name, phone number and voice message transcription
function extractVoicemail_(msg) {
var result = {
'Message Date': msg.getDate(),
'Message Subject': msg.getSubject(),
'Message Body': msg
.getPlainBody()
.replace(/<[^>]+>/g, '')
.replace(/\s+/g, ' '),
Transcription: msg.getPlainBody(),
};
var trans = /transcript:(.*)?play message/i.exec(result['Message Body']);
if (trans) result.Transcription = trans[1];
//Voicemail from: John Q Public (202) 123-456 at 6:08 PM
var match = /(Missed Call|Voicemail) from:([*\+\s\w]+)([\d\-\s\(\)\+]*)? at [\d\:\s]+[ap]m/i.exec(
result['Message Body']
);
if (match) {
result['Call Type'] = match[1];
result['Contact Name'] = match[2].replace(/^+/, '');
result['Contact Number'] = match[3].replace(/^+/, '');
} else {
return null;
}
return result;
}
// Create a new Google contact from Voicemail
function createContact_(result) {
var contacts = ContactsApp.getContactsByPhone(result['Contact Number'], ContactsApp.Field.WORK_PHONE);
if (contacts.length > 0) {
return 'Contact Exists';
} else {
var contact = ContactsApp.createContact(result['Contact Name'], result['Contact Name'], result['Call Type']);
contact.addPhone(ContactsApp.Field.WORK_PHONE, result['Contact Number']);
contact.setNotes(result['Transcription']);
return contact.getId();
}
}
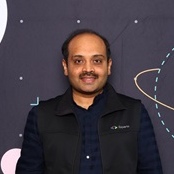
Amit Agarwal
Google Developer Expert, Google Cloud Champion
Amit Agarwal is a Google Developer Expert in Google Workspace and Google Apps Script. He holds an engineering degree in Computer Science (I.I.T.) and is the first professional blogger in India.
Amit has developed several popular Google add-ons including Mail Merge for Gmail and Document Studio. Read more on Lifehacker and YourStory