Create Google Calendar Event with File Attachment
The Google Script will create a new event in the specified Google Calendar and will attach a file from Google Drive into the event.
Google Calendar API only allows file attachments from Google Drive and you can include a maximum of 25 attachment per event. The attachments can be specified either by File ID or by File URL. The Advanced Calendar API should be enabled from your Google Console.
// Credits / References
// https://developers.google.com/google-apps/calendar/v3/reference/events
// http://stackoverflow.com/questions/34853043
function createEvent() {
var calendarId = '{{Google Calendar Id}}';
// April 20, 2016 10:00:00 AM
var start = new Date(2016, 3, 20, 10, 0, 0);
// April 20, 2016 10:30:00 AM
var end = new Date(2016, 3, 20, 10, 30, 0);
var fileName = 'Appraisal Guidlines.pdf';
// Get the Drive ID of the file attachments
// Only Google Drive file are support in Google Calendar
var fileId = DriveApp.getFilesByName(fileName).next().getId();
var calendarEvent = {
summary: 'Performance Appraisal',
description: 'Submit Appraisal Document for March.',
location: '10 Hanover Square, NY 10005',
start: {
dateTime: start.toISOString(),
},
end: {
dateTime: end.toISOString(),
},
attachments: [
{
fileId: fileId,
title: fileName,
},
],
attendees: [
{
email: 'employee1@ctrlq.org',
},
{
email: 'employee2@labnol.org',
},
],
};
// Set supportsAttachments to true
// if the calendarEvent object has one or more file attachments
calendarEvent = Calendar.Events.insert(calendarEvent, calendarId, {
supportsAttachments: true,
});
Logger.log('Event with attachment created. Event ID is %s' + calendarEvent.getId());
// For debugging the output
Logger.log(calendarEvent);
}
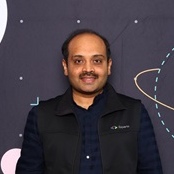
Amit Agarwal
Google Developer Expert, Google Cloud Champion
Amit Agarwal is a Google Developer Expert in Google Workspace and Google Apps Script. He holds an engineering degree in Computer Science (I.I.T.) and is the first professional blogger in India.
Amit has developed several popular Google add-ons including Mail Merge for Gmail and Document Studio. Read more on Lifehacker and YourStory