How to Convert Image to Base64 Data URI
How to convert an image to a base64 data URI with Google Apps Script or the HTML5 Canvas API.
In Spreadsheet Paintings, you upload a photograph from the local disk and it transforms the picture into pixel art. Internally, the JavaScript resizes the image using the HTML5 Canvas API and then uploads the base64-encoded representation of the canvas data to the Google Script using the HTMLService where the pixels are converted into RGB colors.
Image to Base64 with Google Apps Script
const convertImageToDataUri = () => {
const imageUrl = 'https://i.imgur.com/6rl9Atu.png';
const blob = UrlFetchApp.fetch(imageUrl).getBlob();
const base64String = Utilities.base64Encode(blob.getBytes());
return `data:image/png;base64,${base64String}`;
};
Base64 Image with HTML5 Canvas API
<input type="file" id="image" />
<canvas></canvas>
<script>
$('document').ready(function () {
$('input[type=file]').on('change', function (f) {
var file = f.target.files[0];
if (file) {
var reader = new FileReader();
var image = new Image();
reader.onload = function (e) {
image.src = e.target.result;
var canvas = $('canvas')[0];
canvas.height = image.height;
canvas.width = image.width;
var ctx = canvas.getContext('2d');
ctx.drawImage(image, 0, 0);
var dataURL = canvas.toDataURL('image/png');
console.log(dataURL);
};
reader.readAsDataURL(file);
}
});
});
</script>
Embed Base64 Data URI in HTML
Take the base64 string and add it the the src
attribute of an img
tag.
<img src="data:image/png;base64,iVBORw0KGg....." />
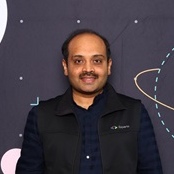
Amit Agarwal
Google Developer Expert, Google Cloud Champion
Amit Agarwal is a Google Developer Expert in Google Workspace and Google Apps Script. He holds an engineering degree in Computer Science (I.I.T.) and is the first professional blogger in India.
Amit has developed several popular Google add-ons including Mail Merge for Gmail and Document Studio. Read more on Lifehacker and YourStory