Get List of Google Team Drives with Apps Script
This Google Apps Script returns a list of Team Drives that the authorized user is part of. The code is written in ES6 and you would need to transpile the code using Babel before pushing it via Google Clasp.
const makeQueryString = (url, params = {}) => {
const paramString = Object.keys(params)
.map((key) => `${encodeURIComponent(key)}=${encodeURIComponent(params[key])}`)
.join('&');
return url + (url.indexOf('?') >= 0 ? '&' : '?') + paramString;
};
const makeHttpGetRequest = (apiUrl, params, accessToken) => {
const url = makeQueryString(apiUrl, params);
const response = UrlFetchApp.fetch(url, {
headers: {
Authorization: `Bearer ${accessToken}`,
},
muteHttpExceptions: true,
});
return JSON.parse(response);
};
const getTeamDrivesForUser = () => {
const params = {
pageSize: 100,
useDomainAdminAccess: true,
};
const data = [];
const accessToken = ScriptApp.getOAuthToken();
const API = 'https://www.googleapis.com/drive/v3/teamdrives';
do {
let response = makeHttpGetRequest(API, params, accessToken);
if (response.teamDrives) {
response.teamDrives.forEach((td) => {
data.push([td.id, td.name]);
});
}
params.pageToken = response.nextPageToken || null;
} while (params.pageToken);
Logger.log(data);
};
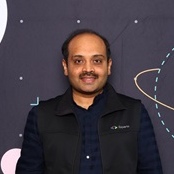
Amit Agarwal
Google Developer Expert, Google Cloud Champion
Amit Agarwal is a Google Developer Expert in Google Workspace and Google Apps Script. He holds an engineering degree in Computer Science (I.I.T.) and is the first professional blogger in India.
Amit has developed several popular Google add-ons including Mail Merge for Gmail and Document Studio. Read more on Lifehacker and YourStory