Google OAuth 2.0 Service account with PHP and Google APIs
This sample code uses Google Service Accounts with PHP to get a list of users in a Google Apps Domain using the Admin Directory API.
To get started, install the Google API PHP library, create a Google Service account with domain-wide delegation and save the private JSON file in the same directory as your PHP application.
<?
require_once __DIR__ . '/vendor/autoload.php';
define('APPLICATION_NAME', 'Service Accounts Tutorial');
define('SCOPES', implode(' ', array(
Google_Service_Drive::DRIVE,
Google_Service_Gmail::MAIL_GOOGLE_COM,
Google_Service_Directory::ADMIN_DIRECTORY_USER_READONLY,
Google_Service_People::USERINFO_EMAIL
)));
putenv('GOOGLE_APPLICATION_CREDENTIALS=private_key.json');
date_default_timezone_set("Asia/India");
$user = "amit@labnol.org";
$client = new Google_Client();
$client->useApplicationDefaultCredentials();
$client->setApplicationName(APPLICATION_NAME);
$client->setScopes(SCOPES);
$client->setAccessType("offline");
$client->setSubject($user);
$service = new Google_Service_Directory($client);
$optParams = array(
'domain' => 'ctrlq.org',
'maxResults' => 10,
'orderBy' => 'email'
);
$results = $service->users->listUsers($optParams);
print_r($results);
?>
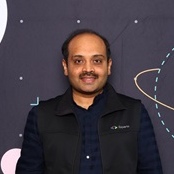
Amit Agarwal
Google Developer Expert, Google Cloud Champion
Amit Agarwal is a Google Developer Expert in Google Workspace and Google Apps Script. He holds an engineering degree in Computer Science (I.I.T.) and is the first professional blogger in India.
Amit has developed several popular Google add-ons including Mail Merge for Gmail and Document Studio. Read more on Lifehacker and YourStory